Vector Multiplication#
Vector multiplication can be performed in three ways:
Scalar Multiplication
Dot Product Multiplication
Cross Product Multiplication
Scalar Multiplication#
Let’s start with scalar multiplication - in other words, multiplying a vector by a single numeric value.
Suppose I want to multiply my vector by 2, which I could write like this:
Note that the result of this calculation is a new vector named w. So how would we calculate this? Recall that v is defined like this:
To calculate 2v, we simply need to apply the operation to each dimension value in the vector matrix, like this:
Which gives us the following result:
In Python, you can apply these sort of matrix operations directly to numpy arrays, so we can simply calculate w like this:
%matplotlib inline
import numpy as np
import matplotlib.pyplot as plt
import math
v = np.array([2,1])
w = 2 * v
print(w)
# Plot w
origin = [0], [0]
plt.grid()
plt.ticklabel_format(style='sci', axis='both', scilimits=(0,0))
plt.quiver(*origin, *w, scale=10)
plt.show()
[4 2]
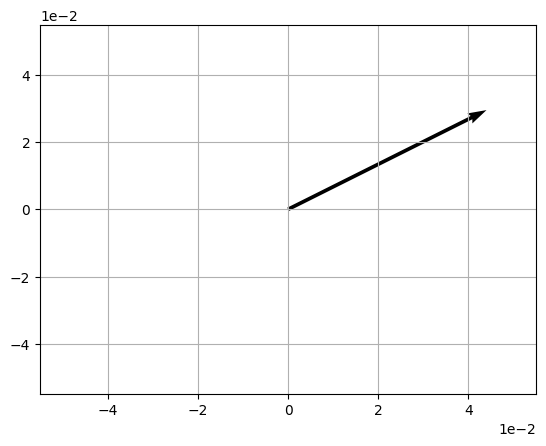
The same approach is taken for scalar division.
Try it for yourself - use the cell below to calculate a new vector named b based on the following definition:
b = v / 2
print(b)
# Plot b
origin = [0], [0]
plt.axis('equal')
plt.grid()
plt.ticklabel_format(style='sci', axis='both', scilimits=(0,0))
plt.quiver(*origin, *b, scale=10)
plt.show()
[1. 0.5]
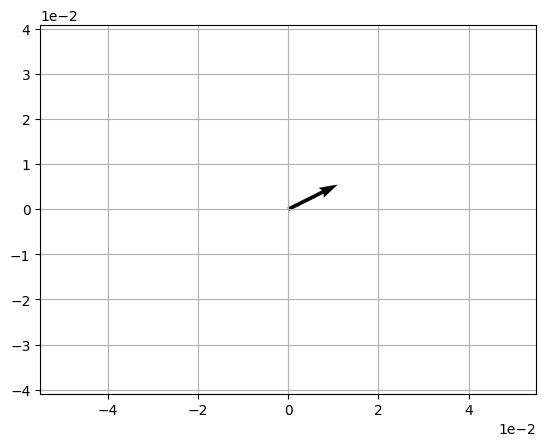
Dot Product Multiplication#
So we’ve seen how to multiply a vector by a scalar. How about multiplying two vectors together? There are actually two ways to do this depending on whether you want the result to be a scalar product (in other words, a number) or a vector product (a vector).
To get a scalar product, we calculate the dot product. This takes a similar approach to multiplying a vector by a scalar, except that it multiplies each component pair of the vectors and sums the results. To indicate that we are performing a dot product operation, we use the • operator:
So for our vectors v (2,1) and s (-3,2), our calculation looks like this:
So the dot product, or scalar product, of v • s is -4.
In Python, you can use the numpy.dot function to calculate the dot product of two vector arrays:
import numpy as np
v = np.array([2,1])
s = np.array([-3,2])
d = np.dot(v,s)
print (d)
-4
In Python 3.5 and later, you can also use the @ operator to calculate the dot product:
import numpy as np
v = np.array([2,1])
s = np.array([-3,2])
d = v @ s
print (d)
-4
The Cosine Rule#
An useful property of vector dot product multiplication is that we can use it to calculate the cosine of the angle between two vectors. We could write the dot products as:
Which we can rearrange as:
So for our vectors v (2,1) and s (-3,2), our calculation looks like this:
So:
Which calculates to:
So:
Here’s that calculation in Python:
import math
import numpy as np
# define our vectors
v = np.array([2,1])
s = np.array([-3,2])
# get the magnitudes
vMag = np.linalg.norm(v)
sMag = np.linalg.norm(s)
# calculate the cosine of theta
cos = (v @ s) / (vMag * sMag)
# so theta (in degrees) is:
theta = math.degrees(math.acos(cos))
print(theta)
119.74488129694222
Cross Product Multiplication#
To get the vector product of multipying two vectors together, you must calculate the cross product. The result of this is a new vector that is at right angles to both the other vectors in 3D Euclidean space. This means that the cross-product only really makes sense when working with vectors that contain three components.
For example, let’s suppose we have the following vectors:
To calculate the cross product of these vectors, written as p x q, we need to create a new vector (let’s call it r) with three components (r1, r2, and r3). The values for these components are calculated like this:
So in our case:
In Python, you can use the numpy.cross function to calculate the cross product of two vector arrays:
import numpy as np
p = np.array([2,3,1])
q = np.array([1,2,-2])
r = np.cross(p,q)
print (r)
[-8 5 1]